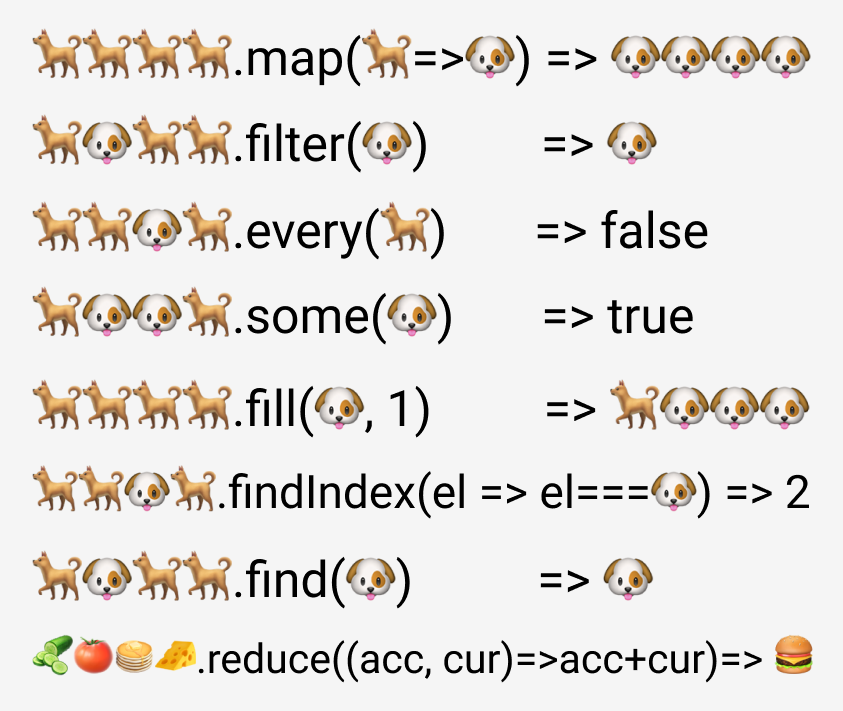
JavaScript array has many build-in methods. These methods are attached to Array's prototype, which we can access by applying the dot operator.
Example:-
map
method must have been attached to Array as
Array.prototype.map = function(){ ......... }
These array methods accept a callback function. Most of these Callback functions accept three parameters. 1st one is the array item(ith element), 2nd one is the index of the item, and the 3rd one is the array itself. All these three parameters are optional.
NOTE: I will use array
keyword to denote a javascript array.
array.map()
array.map
is a JavaScript array method. It iterates over the array and returns a new array. You can pass a callback function to the map method. Callback
function can return the item itself or you can write some logic to alter the item and return a new one.
var arr = [1,2,3,4]; function callback(item,index, arr){ return item; } var res = arr.map(callback); console.log(res) // output = [1,2,3,4] const square = (item) => item * item var squareArr = arr.map(square); console.log(squareArr) // output = [1,4,9,16]
array.filter()
array.filter
is a JavaScript array method. It iterates over the array and returns filtered items. It takes a callback function where you
can write the condition to filter the array.
var arr = [1,2,3,4,5]; function getEvenNo(item, index, arr){ return item % 2 ===0; } var evenArr = arr.filter(getEvenNo) console.log(evenArr) // output = [2,4] const getOddNo = (item, index, arr) => item % 2 !==0; var oddArr = arr.filter(square); console.log(oddArr) // output = [1,3,5];
array.every()
array.every
is a JavaScript array method. It iterates over the array and returns a boolean value. It takes a callback function where you
test each item with the given condition. If all the array items pass the condition, it returns true or else returns false.
var arr = [1,2,3,4,5]; function isAllItemEven(item, index, arr){ return item % 2 ===0; } var isEvenArr = arr.every((item, index, arr) => item % 2 === 0) console.log(isEvenArr) // output = false var isEvenArr = [2,4,6].every((item)=> item % 2 === 0); console.log(isEvenArr) // output = true
array.some()
array.some
is a JavaScript array method. It iterates over the array and returns a boolean value. It takes a callback function
where you test each item with the given condition. As the name suggests, if at least one of the items passes the condition, it returns true or else returns false.
var containsEven = [2,3,6,7].some((item)=> item % 2 ===0 ); console.log(containsEven) // output = true var containsZero = [2,0,6,7].some((item)=> item === 0 ); console.log(containsZero) // output = true
array.fill()
array.fill
is a JavaScript array method. It iterates over the array and return a new array. The fill()
method
changes all elements in an array to a static value
arr.fill(value, start, end)
As u can see it has 3 parameters.
value (1st paramenter)- It replace all the array items with value.
start (2nd paramenter)- It is the starting point. From this index, each array item will be replaced with the value.
end (3rd paramenter)- It is the endpoint or the end of the index. No further element will be replaced with the value after this.
If you do not pass the first paramenter value, then array will return all the items as
undefined
var array = [1, 2, 3, 4, 5]; array.fill(0) // Output: [0, 0, 0, 0, 0] var array = [1, 2, 3, 4, 5]; array.fill(0,2) // Output: [1, 2, 0, 0, 0] var array = [1, 2, 3, 4, 5]; array.fill(0,2,4) // Output: [1, 2, 0, 0, 5] var array = [1, 2, 3, 4]; array.fill() // Output: [undefined, undefined, undefined, undefined]
array.fill
method can be used to initialised an array with some default value.For Example - You are working on an algorithm, and you want an array of size n, where each item is initialized with -1.
var n = 100; new Array(n).fill(-1) // it will create an aryay having length 100 // with each cell initialised with value -1
array.findIndex()
array.findIndex
is a JavaScript array method. It is used to find the index of an item. It returns the index of the found item or returns -1.
It takes a callback function where you can write your logic.
var arr = [1,2,3,4,5]; arr.findIndex(item => item === 3) // Output: 2 var arr = [{id: 0, num: 0},{id: 1, num: 1},{id: 2, num: 2},{id: 3, num: 3},{id: 4, num: 4}]; function find(item){ return item.id === 2 } arr.findIndex(find) // output = 2 var arr = [{id: 0, num: 0},{id: 1, num: 1},{id: 2, num: 2},{id: 3, num: 3},{id: 4, num: 4}]; function find(item){ return item.id === 8 } arr.findIndex(find) // output = -1
array.find()
array.find
is a JavaScript array method. It is very much similar to findIndex. However, It returns the found item instead of its index.
It takes a callback function where you can write your logic. It returns the found item or returns undefined.
var arr = [1,2,3,4,5]; arr.find(item => item === 2) // Output: 2 var arr = [{id: 0, num: 0},{id: 1, num: 1},{id: 2, num: 2},{id: 3, num: 3}]; function findItem(item){ return item.id === 2 } arr.find(findItem) // output = {id: 2, num: 2} var arr = [{id: 0, num: 0},{id: 1, num: 1},{id: 2, num: 2},{id: 3, num: 3}]; function findItem(item){ return item.id === 8 } arr.find(findItem) // output = undefined
array.reduce()
array.reduce
is a JavaScript array method. It iterates over the array items and returns a single output value.
It is a very interesting array method that can be used in many ways. array.reduce
methods takes 2 arguments- a callback function
and an initialValue
The callback function takes four arguments: Accumulator, currentValue, index and sourceArray
The accumulator value is remembered on each iteration. Current Value is the current item of iteration.
array.reduce((accumulator, currentValue, index, array) => { //your logic }, initialValue) [1,2,3,4].reduce((accumulator, currentValue) =>{ return accumulator + currentValue; }, 0) // Output: 10
Accumulator set with returned value after each iteration. In the above example, the accumulator is initialized with 0 but get a new value on each iteration.
Let's see another example to understand it better way.
[1, 40, 45, 55, 105, 100].reduce((accumulator, curValue) => { return Math.max(accumulator, curValue) }, 50); //Output: 105
In the above example, each iteration returns the max value between the accumulator and the currentValue- Math.max(accumulator, curValue). The returned max value replaces the old accumulator value. This way we get the max value from the array.
0 Comments