A frontend developer mostly use console.log()
and at max console.error()
. As a frontend dev,
I never cared about console, as I was hardly using any of its feature except console.log()
. But, do you know there are a
lot of console methods which we can use. We can use these different methods to format, style, calculate time and inform the user about
the type of message we are logging.
Before diving into the topic, let's understand what is console?
console
is a JavaScript object which has lots of methods to log the messages on the console. It provides the access to the browser's debugging
console. All the console methods may not be available in all the browser but, there are a generic set of features that are available.
Below is the list of methods present in console object:-

NOTE: Most of the methods are available in all browsers except few.
console.trace
console.trace
is used to trace the calls sequentially. In other words, the call that happened first is at the bottom of the stack trace.
You can log stack traces at any time by calling console.trace()
.
function main(){ subFun(); } function subFun(){ console.trace('Trace main function'); } main()

console.table
console.table
prints a data in tabular format. it takes an array as an argument and print all the items in the value column.
console.table(["John", "Dev", "Sid"]);

We can also pass an object as an argument. Key will be printed in the first column and value will be printed in the 2nd column.
var obj = {fname: "John", lname: "Smith", age: 30}; console.table(obj);

We can also pass array of arrays. In this case, each item of child array will be logged in new column in the same row.
console.table([ ["Alabama", "Alaska"], ["Moscow", "St. Petersburg"], ["Sydney", "Melbourne"] ]);

Note: in Firefox, console.table
is limited to displaying 1000 rows.
console.group
console.group
is one of the important methods, it is used to group messages on the console. After console.group
you need to use console.log
to put a message within that group. you can create nesting of groups. You can come out of a group
by using console.groupEnd
. You can expand or collapse a group by clicking the arrow icon.
console.log("This is the outer level"); console.group("America"); console.group("Alabama"); console.log("Birmingham"); console.groupEnd(); console.group("Alaska"); console.log("Anchorage"); console.groupEnd(); console.groupEnd();

Timers
Javascript console has timers methods to log the time consumed between the lines of code. It has a combination of methods to achieve that.
For example- Let say, we want to calculate the time taken for an array operation. We can use console.time
with one paramenter (string)
and console.timeEnd
with same parameter.
console.time("Array operation"); var arr = []; for(var i = 0; i < 100000; i++) { arr.push(Math.random()); } console.timeLog("Array operation"); console.timeEnd("Array operation");

NOTE: console.timeLog
logs the time taken for the operation, so does console.timeEnd
. But, console.timeEnd
ends the timer. If you do not use console.timeEnd
then timer will keep running.
Let's take another example and calculate the time taken for an api call.
console.time("api call"); var res = await fetch('https://jsonplaceholder.typicode.com/posts').then(res => res.json()); console.timeEnd("api call");

NOTE: Timers will report the total time for the transaction, while the time listed in the network panel is just the amount of time required for the header.
console.dir
console.dir
is used to log javascript objects. The output is presented as a hierarchical listing with disclosure triangles
that let you see the contents of child objects.
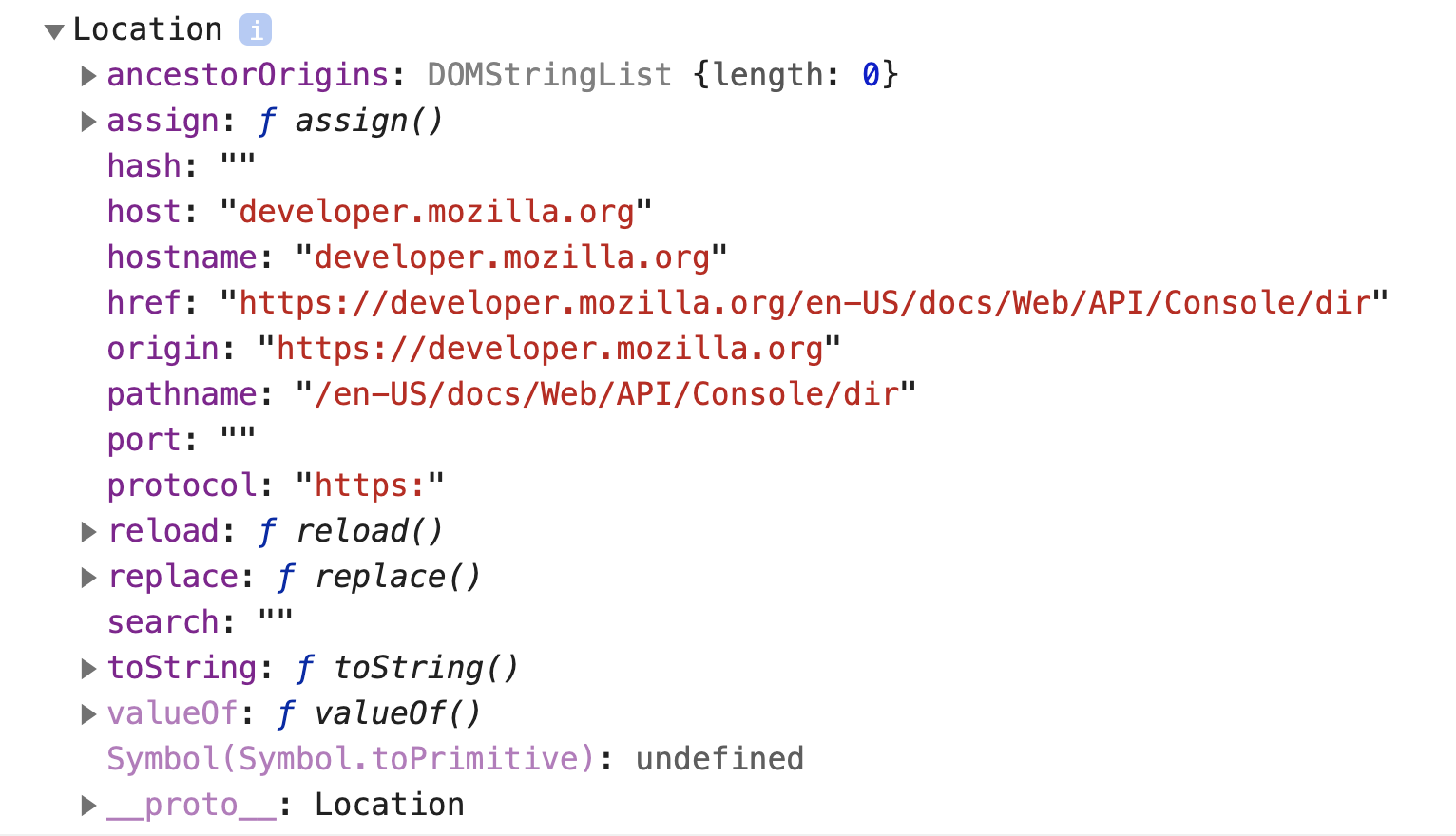
console.warn
console.warn
is used to log warning message.
console.warn("This module is deprecated, please use latest one");

console.error
console.error
is used to log error message. When you handle exception in JavaScript, it is good to use console.error
for
showig error messages.
Let say, below api fails due to some reason, in that case it will log the error message.
fetch("http://user.api.com/getusers") .then(function() { console.log("ok"); }).catch(function(error) { console.error("Failed to get users data"); });

How to style console logs in javascript ?
Do you know, you can style the console output 🤔?
Yes, It is possible. We can use css style to style cosole logs. Have you enter inspected facebook in connsole. Just go to facebook ad opne the developer tool. You can see that console messages are styled. They use css style to style the logs.
console.log('%cWarning', `color: red; font-size:30px;`); console.log('%cBe aware of XSS attacks', `color: orange; font-size:16px;`); console.log('%cPlease do not try to use any malicious script', `color: #333; font-size:12px;`);

String substitutions in javascript- How to pass dynamic values to console.log ?
%s
%s
is used to output string.
var film = "Gladiator"; var actor1 = "Russell Crowe"; var actor2 = "Connie Nielsen"; console.log("Film %s has 2 actors %s and %s", film, actor1, actor2);

%o or %O
%o or %O
is used to outputs object.
var wrestler = {name: "John Cena", age: 35} console.log("WWE fighter %o is holdinng the top rank currently", wrestler);

%d or %i
%d or %i
is used to outputs Number.
console.log("Marks in Math = %d", 78); console.log("Marks in Physics = %i", 55);

%f
%f
is used to outputs decimal number.
console.log("Todays temerature is %f degree celsius", 38.53);

0 Comments